Posts tagged jQuery
Creating photo gallery using jQuery and VisualLightBox
- 25
- Jul
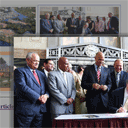
Creating photo gallery using jQuery and VisualLightBox
Today I will tell you about one useful JQuery plugin – VisualLightBox.
This plugin can help you to create good-looking galleries. And this is very easy to implement.
Read more
Import RSS Feeds using jFeed (jQuery)
- 01
- Jun
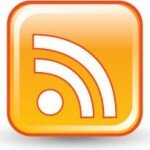
RSS feed – using jFeed (jQuery) to aggregate RSS
This simple tutorial will show you how to Import rss feeds of any site into your own custom area/block of website. It can be used as news imported from yahoo, bbc etc. As we know, RSS feeds are usually used to trasnfer some news for people. Today I’ll tell you about RSS feeds and show you how to make an own RSS aggregator. In the result, you will be able to display various RSS feeds on your website with nite and modern design.
Here is the sample:
Live Demo
download in package
Well, download the source files and let’s start coding !
Step 1. HTML
As usual, we start with the HTML.
This is our main page code (with 2 RSS blocks). Pay heed at RSSAggrCont elements. Param ‘rssnum’ means – how many rss elements will be displayed at the page. ‘rss_url’ – is RSS url. I hope that you will be able to change these params easily.
templates/rss_page.html
<link href="templates/css/styles.css" rel="stylesheet" type="text/css" /> <script language="javascript" type="text/javascript" src="js/jquery-1.4.2.min.js"></script> <script language="javascript" type="text/javascript" src="js/jquery.jfeed.js"></script> <script language="javascript" type="text/javascript" src="js/jquery.aRSSFeed.js"></script> <div class="RSSAggrCont" rssnum="5" rss_url="http://rss.news.yahoo.com/rss/topstories"> <div class="loading_rss"> <img alt="Loading..." src="templates/images/loading.gif" /> </div> </div> <div class="RSSAggrCont" rssnum="5" rss_url="http://newsrss.bbc.co.uk/rss/newsonline_world_edition/front_page/rss.xml"> <div class="loading_rss"> <img alt="Loading..." src="templates/images/loading.gif" /> </div> </div> <script language="javascript" type="text/javascript"> $(document).ready( function() { $('div.RSSAggrCont').aRSSFeed(); } ); </script>
Step 2. CSS
Here are CSS styles.
templates/css/styles.css
.RSSAggrCont { border:1px solid #AAA; -moz-box-shadow:0 0 10px #ccc; -webkit-box-shadow: 0 0 10px #ccc; width:500px; padding:10px; background:#f3f3f3; margin:15px; float:left; } /* RSS Feeds */ .rss_item_wrapper { padding-bottom: 8px; } .rss_item_wrapper:last-child { padding-bottom: 0px; } .rss_item_header { font-size:12px; font-weight:bold; padding-bottom:0px; } .rss_item_header a, .rss_item_header a:link, .rss_item_header a:visited, .rss_item_header a:hover, .rss_item_header a:active { font-size:12px; font-weight:bold; color:#33c; } .rss_item_info { color:#999; font-size:9px; } .rss_item_desc { text-align:justify; font-size: 11px; } .rss_read_more { background-color:#EDEDED; font-size:11px; font-weight:normal; height:30px; line-height:30px; vertical-align: middle; margin-top:2px; padding:0 9px; text-align:left; text-decoration:none; text-transform:capitalize; } .loading_rss { text-align:center; width:89px; height:64px; background-image:url(../images/loading_bg.png); z-index: 10; margin: 10px auto; } .loading_rss img { margin-top: 16px; } .loading_rss div { width:89px; height:64px; background-image:url(../images/loading.gif); background-position:center center; background-repeat:no-repeat; }
Step 3. JS
Here are few necessary JS files for our project:
js/jquery.aRSSFeed.js
// jQuery plugin - Dolphin RSS Aggregator (function($){ $.fn.aRSSFeed = function() { return this.each( function(){ var $Cont = $(this); var iMaxNum = parseInt($Cont.attr( 'rssnum' ) || 0); var sFeedURL = $Cont.attr('rss_url'); if (sFeedURL == undefined) return false; $.getFeed ({ url: 'get_rss_feed.php?url=' + escape(sFeedURL), success: function(feed) { if (feed != undefined && feed.items) { var sCode = '<div class="rss_feed_wrapper">'; var iCount = 0; for (var iItemId = 0; iItemId < feed.items.length; iItemId ++) { var item = feed.items[iItemId]; var sDate; var a; var oDate if (null != (a = item.updated.match(/(\d+)-(\d+)-(\d+)T(\d+):(\d+):(\d+)Z/))) oDate = new Date(a[1], a[2]-1, a[3], a[4], a[5], a[6], 0); else oDate = new Date(item.updated); sDate = oDate.toLocaleString(); sCode += '<div class="rss_item_wrapper">' + '<div class="rss_item_header">' + '<a href="' + item.link + '" target="_blank">' + item.title + '</a>' + '</div>' + '<div class="rss_item_info">' + '<span><img src="templates/images/clock.png" /> ' + sDate + '</span>' + '</div>' + '<div class="rss_item_desc">' + item.description + '</div>' + '</div>'; iCount ++; if (iCount == iMaxNum) break; } sCode += '</div>' + '<div class="rss_read_more">' + '<img class="bot_icon_left" src="templates/images/more.png" />' + '<a href="' + feed.link + '" target="_blank" class="rss_read_more_link">' + feed.title + '</a>' + '</div>' + '<div class="clear_both"></div>'; $Cont.html(sCode); } } } ); } ); }; })(jQuery);
js/jquery-1.4.2.min.js and js/jquery.jfeed.js
This are common files – jQuery library with jFeed library. It is to no purpose to publish full codes of these files here (they are pretty huge). Both are available in our download package
Step 4. PHP
Finally – PHP sources, I think that everything should be easy to understand:
index.php
<?php require_once('templates/rss_page.html'); ?>
get_rss_feed.php
<?php $sUrl = (isset($_GET['url']) && $_GET['url'] != '') ? $_GET['url'] : 'http://www.boonex.com/unity/extensions/latest/?rss=1'; header( 'Content-Type: text/xml' ); readfile($sUrl); ?>
Step 5. Images
Several images are required for our project:
Live Demo
download in package
Conclusion
Today I’ve described you how to create own RSS aggregator using jQuery library – jFeed. You are welcome to use it in your projects. Good luck!
How to Easily Make AJAX Style PHP Chat Application
- 18
- May
How to Easily Make “Ajaxy” Chat application with PHP + SQL + jQuery
Today I will tell you about another chat system. After making old-style chat described in previous post I decided to make modern, pretty version. I made several enhancements:
- a) Login system. Now it using database to keep members. Also it have easy methods to take some necessary info about logged member.
- b) Chat interface. Now it much more user friendly. And show last added messages via toggling (based on jQuery). Looks fine.
- c) Inner code structure. Now here are good order. All libraries in ‘inc’ folder, all javascripts in ‘js’ folder, all template-related files in folder ‘templates’. More, class organization: now we have useful DB class, class of login system, chat class too.
- d) of code safety. Added necessary methods to protect from hack attacks. As example function process_db_input in our DB class.
So, as result – we will able to chat with our logged members. We will use database to store messages as usual.
Read more
Advance Level Php Tutorials and Scripts
- 26
- Mar
Advance Level Php Tutorials and Scripts
Some pretty cool and amazing working php scripts with sources and tutorials for you to use on your sites. Some are Tutorials for security, some are images manipulation techniques while some are really high level plugins like sponsor flips, jquery sorting and vote poll etc. Hope you find it Useful !
All Tutorials are properly linked back to their original sources.
Jquery CSS Tutorial on Zooming Image
- 15
- Mar
Jquery CSS Tutorial on how to Zoom Images
Today I will tell you about another one useful tool of JQuery – Zoomimage plugin. It allow to reach different design ideas with images resizing.